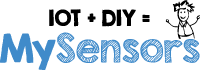 |
MySensors Library & Examples
2.3.2
|
Go to the documentation of this file.
39 #define V2_MYS_HEADER_PROTOCOL_VERSION (2u)
40 #define V2_MYS_HEADER_SIZE (7u)
41 #define V2_MYS_HEADER_MAX_MESSAGE_SIZE (32u)
43 #define V2_MYS_HEADER_VSL_VERSION_POS (0)
44 #define V2_MYS_HEADER_VSL_VERSION_SIZE (2u)
45 #define V2_MYS_HEADER_VSL_SIGNED_POS (2u)
46 #define V2_MYS_HEADER_VSL_SIGNED_SIZE (1u)
47 #define V2_MYS_HEADER_VSL_LENGTH_POS (3u)
48 #define V2_MYS_HEADER_VSL_LENGTH_SIZE (5u)
50 #define V2_MYS_HEADER_CEP_COMMAND_POS (0)
51 #define V2_MYS_HEADER_CEP_COMMAND_SIZE (3u)
52 #define V2_MYS_HEADER_CEP_ECHOREQUEST_POS (3u)
53 #define V2_MYS_HEADER_CEP_ECHOREQUEST_SIZE (1u)
54 #define V2_MYS_HEADER_CEP_ECHO_POS (4u)
55 #define V2_MYS_HEADER_CEP_ECHO_SIZE (1u)
56 #define V2_MYS_HEADER_CEP_PAYLOADTYPE_POS (5u)
57 #define V2_MYS_HEADER_CEP_PAYLOADTYPE_SIZE (3u)
59 #define MAX_MESSAGE_SIZE V2_MYS_HEADER_MAX_MESSAGE_SIZE
60 #define HEADER_SIZE V2_MYS_HEADER_SIZE
61 #define MAX_PAYLOAD_SIZE (MAX_MESSAGE_SIZE - HEADER_SIZE)
64 #define MAX_PAYLOAD MAX_PAYLOAD_SIZE
78 #if !DOXYGEN // Hide until we migrate
100 S_ARDUINO_REPEATER_NODE = 18,
107 S_SCENE_CONTROLLER = 25,
122 } mysensors_sensor_t;
149 V_HVAC_FLOW_STATE = 21,
173 V_HVAC_SETPOINT_COOL = 44,
174 V_HVAC_SETPOINT_HEAT = 45,
175 V_HVAC_FLOW_MODE = 46,
253 #define BIT(n) ( 1<<(n) )
255 #define BIT_MASK(len) ( BIT(len)-1 )
256 #define BF_MASK(start, len) ( BIT_MASK(len)<<(start) )
258 #define BF_PREP(x, start, len) ( ((x)&BIT_MASK(len)) << (start) )
259 #define BF_GET(y, start, len) ( ((y)>>(start)) & BIT_MASK(len) )
260 #define BF_SET(y, x, start, len) ( y= ((y) &~ BF_MASK(start, len)) | BF_PREP(x, start, len) )
264 #define mSetVersion(_message, _version) _message.setVersion(_version)
265 #define mGetVersion(_message) _message.getVersion()
267 #define mSetSigned(_message, _signed) _message.setSigned(_signed)
268 #define mGetSigned(_message) _message.getSigned()
270 #define mSetLength(_message,_length) _message.setLength(_length)
271 #define mGetLength(_message) _message.getLength()
273 #define mSetCommand(_message, _command) _message.setCommand(_command)
274 #define mGetCommand(_message) _message.getCommand()
276 #define mSetRequestEcho(_message, _requestEcho) _message.setRequestEcho(_requestEcho)
277 #define mGetRequestEcho(_message) _message.getRequestEcho()
279 #define mSetEcho(_message, _echo) _message.setEcho(_echo)
280 #define mGetEcho(_message) _message.getEcho()
282 #define mSetPayloadType(_message, _payloadType) _message.setPayloadType(_payloadType)
283 #define mGetPayloadType(_message) _message.getPayloadType()
285 #if defined(__cplusplus) || defined(DOXYGEN)
292 char* getCustomString(
char *buffer)
const;
306 MyMessage(
const uint8_t sensorId,
const mysensors_data_t dataType);
360 int16_t
getInt(
void)
const;
480 bool isAck(
void)
const;
559 MyMessage&
set(
const void* payload,
const size_t length);
566 #if !defined(__linux__)
579 MyMessage&
set(
const float value,
const uint8_t decimals);
662 #if defined(__cplusplus) || defined(DOXYGEN)
@ I_POST_SLEEP_NOTIFICATION
Message sent after node woke up (if enabled)
uint8_t sender
8 bit - Id of sender node (origin)
@ I_INCLUSION_MODE
Inclusion mode.
@ I_LOG_MESSAGE
Log message.
@ I_BATTERY_LEVEL
Battery level.
mysensors_stream_t
Type of data stream (for streamed message)
int16_t getInt(void) const
Get signed 16-bit integer payload.
MyMessage & setSensor(const uint8_t sensorId)
Set which child sensor this message belongs to.
@ I_NONCE_REQUEST
Request for a nonce.
@ ST_FIRMWARE_RESPONSE
Response FW block.
mysensors_internal_t
Type of internal messages (for internal messages)
#define MAX_PAYLOAD_SIZE
The maximum size of a payload depends on MAX_MESSAGE_SIZE and HEADER_SIZE.
uint8_t fPrecision
Number of decimals when serializing.
@ ST_FIRMWARE_RESPONSE_RLE
Response FW block with run length encoded data.
const char * getString(void) const
Get payload as string.
uint8_t getSensor(void) const
Get sensor ID of message.
@ I_NONCE_RESPONSE
Payload is nonce data.
@ I_CONFIG
Config (request/response)
@ C_INTERNAL
Internal MySensors messages (also include common messages provided/generated by the library).
uint8_t getExpectedMessageSize(void) const
getExpectedMessageSize
@ I_LOCKED
Node is locked (reason in string-payload)
float fValue
< Float messages
@ I_SIGNAL_REPORT_REVERSE
Internal.
void * getCustom(void) const
Get custom payload.
MyMessage & setSender(const uint8_t senderId)
Set sender ID.
@ P_ULONG32
Payload type is UINT32.
uint8_t getMaxPayloadSize(void) const
getMaxPayloadSize
mysensors_payload_t getPayloadType(void) const
Getter for payload type.
@ C_REQ
Requests a variable value (usually from an actuator destined for controller).
@ I_REBOOT
Reboot request.
@ I_FIND_PARENT_RESPONSE
Find parent response.
bool isProtocolVersionValid(void) const
isProtocolVersionValid
@ I_DISCOVER_REQUEST
Discover request.
@ I_ID_RESPONSE
ID response.
bool getSigned(void) const
Getter for sign field.
int32_t lValue
signed long value (32-bit)
MyMessage & setPayloadType(const mysensors_payload_t payloadType)
Setter for payload type.
uint8_t getSender(void) const
Get sender ID.
uint8_t last
8 bit - Id of last node this message passed
@ P_FLOAT32
Payload type is float32.
uint32_t getULong(void) const
Get unsigned 32-bit integer payload.
mysensors_command_t
The command field (message-type) defines the overall properties of a message.
@ I_SIGNAL_REPORT_RESPONSE
Device signal strength response (RSSI)
MyMessage & setVersion(void)
Setter for version.
@ P_UINT16
Payload type is UINT16.
int16_t iValue
signed integer value (16-bit)
@ I_HEARTBEAT_REQUEST
Heartbeat request.
mysensors_command_t getCommand(void) const
Getter for command type.
@ I_SKETCH_VERSION
Sketch version.
@ ST_FIRMWARE_REQUEST
Request FW block.
MyMessage & setType(const uint8_t messageType)
Set message type.
@ I_SIGNAL_REPORT_REQUEST
Device signal strength request.
int32_t getLong(void) const
Get signed 32-bit integer payload.
MyMessage & set(const void *payload, const size_t length)
Set entire payload.
struct @4::@5 __attribute__
Doxygen will complain without this comment.
uint16_t getUInt(void) const
Get unsigned 16-bit integer payload.
uint8_t getDestination(void) const
Get destination.
uint8_t command_echo_payload
@ C_INVALID_7
C_INVALID_7.
@ P_STRING
Payload type is string.
uint8_t getHeaderSize(void) const
getHeaderSize
@ ST_FIRMWARE_CONFIG_REQUEST
Request new FW, payload contains current FW details.
@ C_SET
This message is sent from or to a sensor when a sensor value should be updated.
MyMessage & setLength(const uint8_t length)
Setter for length.
uint8_t getType(void) const
Get message type.
MyMessage & setLast(const uint8_t lastId)
Set last ID.
@ ST_FIRMWARE_CONFIRM
Mark running firmware as valid (MyOTAFirmwareUpdateNVM + mcuboot)
@ C_RESERVED_5
C_RESERVED_5.
@ I_FIND_PARENT_REQUEST
Find parent.
@ P_CUSTOM
Payload type is binary.
MyMessage & setEcho(const bool echo)
Setter for echo-flag.
@ I_TIME
Time (request/response)
@ C_RESERVED_6
C_RESERVED_6.
bool isAck(void) const
Getter for echo-flag.
MyMessage & setDestination(const uint8_t destinationId)
Set final destination node id for this message.
uint8_t getByte(void) const
Get unsigned 8-bit integer payload.
@ I_PING
Ping sent to node, payload incremental hop counter.
@ I_ID_REQUEST
ID request.
char data[MAX_PAYLOAD_SIZE+1]
Buffer for raw payload data.
void clear(void)
Clear message contents.
@ I_HEARTBEAT_RESPONSE
Heartbeat response.
uint8_t getLast(void) const
Get last ID.
@ I_PRE_SLEEP_NOTIFICATION
Message sent before node is going to sleep.
MyMessage & setSigned(const bool signedFlag)
Setter for sign field.
@ I_GATEWAY_READY
Gateway ready.
MyMessage & setCommand(const mysensors_command_t command)
Setter for command type.
FW config structure, stored in eeprom.
char * getStream(char *buffer) const
@ ST_FIRMWARE_CONFIG_RESPONSE
New FW details to initiate OTA FW update.
@ P_LONG32
Payload type is INT32.
MyMessage & setRequestEcho(const bool requestEcho)
Setter for echo request.
uint8_t sensor
8 bit - Id of sensor that this message concerns.
float getFloat(void) const
Get float payload.
@ I_PRESENTATION
Presentation message.
@ P_INT16
Payload type is INT16.
@ I_PONG
In return to ping, sent back to sender, payload incremental hop counter.
bool getRequestEcho(void) const
Getter for echo request.
mysensors_payload_t
Type of payload.
uint16_t uiValue
unsigned integer value (16-bit)
bool isEcho(void) const
Getter for echo-flag.
uint8_t getVersion(void) const
Getter for version.
@ I_DISCOVER_RESPONSE
Discover response.
uint8_t type
8 bit - Type varies depending on command
@ I_REGISTRATION_RESPONSE
Register response from GW.
uint8_t bValue
unsigned byte value (8-bit)
uint32_t ulValue
unsigned long value (32-bit)
bool getBool(void) const
Get bool payload.
MyMessage is used to create, manipulate, send and read MySensors messages.
#define HEADER_SIZE
The size of the header.
@ I_SIGNING_PRESENTATION
Provides signing related preferences (first byte is preference version)
uint8_t getLength(void) const
Getter for length.
uint8_t destination
8 bit - Id of destination node
@ C_STREAM
For firmware and other larger chunks of data that need to be divided into pieces.
@ I_REGISTRATION_REQUEST
Register request to GW.
@ I_SKETCH_NAME
Sketch name.
@ P_BYTE
Payload type is byte.
@ C_PRESENTATION
Sent by a node when they present attached sensors. This is usually done in presentation() at startup.