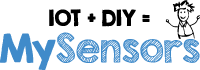 |
MySensors Library & Examples
2.3.2-62-ge298769
|
22 #ifndef EthernetClient_h
23 #define EthernetClient_h
26 #include "IPAddress.h"
29 #define ETHERNETCLIENT_W5100_CLOSED 0x00
30 #define ETHERNETCLIENT_W5100_LISTEN 0x14
31 #define ETHERNETCLIENT_W5100_SYNSENT 0x15
32 #define ETHERNETCLIENT_W5100_SYNRECV 0x16
33 #define ETHERNETCLIENT_W5100_ESTABLISHED 0x17
34 #define ETHERNETCLIENT_W5100_FIN_WAIT 0x18
35 #define ETHERNETCLIENT_W5100_CLOSING 0x1A
36 #define ETHERNETCLIENT_W5100_TIME_WAIT 0x1B
37 #define ETHERNETCLIENT_W5100_CLOSE_WAIT 0x1C
38 #define ETHERNETCLIENT_W5100_LAST_ACK 0x1D
64 virtual int connect(
const char *host, uint16_t port);
79 virtual size_t write(uint8_t b);
87 virtual size_t write(
const uint8_t *buf,
size_t size);
94 size_t write(
const char *str);
102 size_t write(
const char *buffer,
size_t size);
122 virtual int read(uint8_t *buf,
size_t bytes);
132 virtual void flush();
173 virtual operator bool();
180 return bool() == value;
188 return bool() != value;
virtual bool operator!=(const bool value)
Overloaded cast operators.
virtual uint8_t connected()
Whether or not the client is connected.
virtual bool operator!=(const EthernetClient &rhs)
Overloaded cast operators.
void close()
Close the connection.
virtual int peek()
Returns the next byte of the read queue without removing it from the queue.
virtual int connect(const char *host, uint16_t port)
Initiate a connection with host:port.
uint8_t status()
Connection status.
virtual size_t write(uint8_t b)
Write a byte.
virtual void flush()
Waits until all outgoing bytes in buffer have been sent.
int getSocketNumber()
Get the internal socket file descriptor.
void bind(IPAddress ip)
Bind the conection to the specified local ip.
virtual int read()
Read a byte.
EthernetClient()
EthernetClient constructor.
A class to make it easier to handle and pass around IP addresses.
virtual void stop()
Close the connection gracefully.
virtual bool operator==(const bool value)
Overloaded cast operators.
virtual int available()
Returns the number of bytes available for reading.