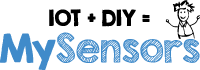 |
MySensors Library & Examples
2.3.2-62-ge298769
|
Go to the documentation of this file.
62 #include "RF24registers.h"
64 #if !defined(RF24_SPI)
65 #define RF24_SPI hwSPI
68 #if defined(ARDUINO_ARCH_AVR)
69 #define DEFAULT_RF24_CE_PIN (9)
70 #elif defined(ARDUINO_ARCH_ESP8266)
71 #define DEFAULT_RF24_CE_PIN (4)
72 #elif defined(ARDUINO_ARCH_ESP32)
73 #define DEFAULT_RF24_CE_PIN (17)
74 #elif defined(ARDUINO_ARCH_SAMD)
75 #define DEFAULT_RF24_CE_PIN (27)
76 #elif defined(LINUX_ARCH_RASPBERRYPI)
77 #define DEFAULT_RF24_CE_PIN (22)
78 //#define DEFAULT_RF24_CS_PIN (24)
79 #elif defined(ARDUINO_ARCH_STM32F1)
80 #define DEFAULT_RF24_CE_PIN (PB0)
81 #elif defined(TEENSYDUINO)
82 #define DEFAULT_RF24_CE_PIN (9)
84 #define DEFAULT_RF24_CE_PIN (9)
87 #define DEFAULT_RF24_CS_PIN (SS)
93 #define RF24_SPI_DATA_ORDER MSBFIRST
94 #define RF24_SPI_DATA_MODE SPI_MODE0
96 #define RF24_BROADCAST_ADDRESS (255u)
99 #if defined(MY_RX_MESSAGE_BUFFER_FEATURE)
100 #if !defined(MY_RF24_IRQ_PIN)
101 #error Message buffering feature requires MY_RF24_IRQ_PIN to be defined!
105 #error RF24 IRQ usage cannot be used with Soft SPI
108 #ifdef ARDUINO_ARCH_ESP8266
109 #error RF24 IRQ usage cannot be used with ESP8266
111 #ifndef SPI_HAS_TRANSACTION
112 #error RF24 IRQ usage requires transactional SPI support
115 #ifdef MY_RX_MESSAGE_BUFFER_SIZE
116 #error Receive message buffering requires RF24 IRQ usage
122 #if defined(MY_RX_MESSAGE_BUFFER_FEATURE)
123 #define RF24_CONFIGURATION (uint8_t) ((RF24_CRC_16 << 2) | (1 << RF24_MASK_TX_DS) | (1 << RF24_MASK_MAX_RT))
125 #define RF24_CONFIGURATION (uint8_t) (RF24_CRC_16 << 2)
127 #define RF24_FEATURE (uint8_t)( _BV(RF24_EN_DPL))
128 #define RF24_RF_SETUP (uint8_t)(( ((MY_RF24_DATARATE & 0b10 ) << 4) | ((MY_RF24_DATARATE & 0b01 ) << 3) | (MY_RF24_PA_LEVEL << 1) ) + 1)
131 #define RF24_POWERUP_DELAY_MS (100u)
134 #define RF24_BROADCAST_PIPE (1u)
135 #define RF24_NODE_PIPE (0u)
157 const bool readMode);
179 #define RF24_readByteRegister(__reg) RF24_RAW_readByteRegister(RF24_CMD_READ_REGISTER | (RF24_REGISTER_MASK & (__reg)))
180 #define RF24_writeByteRegister(__reg,__value) RF24_RAW_writeByteRegister(RF24_CMD_WRITE_REGISTER | (RF24_REGISTER_MASK & (__reg)), __value)
181 #define RF24_writeMultiByteRegister(__reg,__buf,__len) RF24_spiMultiByteTransfer(RF24_CMD_WRITE_REGISTER | (RF24_REGISTER_MASK & (__reg)),(uint8_t *)__buf, __len,false)
239 const bool noACK =
false);
389 #if defined(MY_RX_MESSAGE_BUFFER_FEATURE)
393 typedef void (*RF24_receiveCallbackType)(void);
402 LOCAL void RF24_registerReceiveCallback(RF24_receiveCallbackType cb);
LOCAL void RF24_ce(const bool level)
RF24_ce.
LOCAL void RF24_setRFSetup(const uint8_t RFsetup)
RF24_setRFSetup.
LOCAL bool RF24_getReceivedPowerDetector(void) __attribute__((unused))
Retrieve latched RPD power level, in receive mode (for testing, nRF24L01+ only).
LOCAL void RF24_powerDown(void)
RF24_powerDown.
LOCAL void RF24_powerUp(void)
RF24_powerUp.
LOCAL uint8_t RF24_getDynamicPayloadSize(void)
RF24_getDynamicPayloadSize.
LOCAL void RF24_setFeature(const uint8_t feature)
RF24_setFeature.
LOCAL uint8_t RF24_RAW_readByteRegister(const uint8_t cmd)
RF24_RAW_readByteRegister.
LOCAL uint8_t RF24_getNodeID(void)
RF24_getNodeID.
LOCAL void RF24_enableConstantCarrierWave(void) __attribute__((unused))
Generate a constant carrier wave at active channel & transmit power (for testing only).
LOCAL uint8_t RF24_getStatus(void)
RF24_getStatus.
LOCAL void RF24_stopListening(void)
RF24_stopListening.
LOCAL bool RF24_sendMessage(const uint8_t recipient, const void *buf, const uint8_t len, const bool noACK=false)
RF24_sendMessage.
LOCAL int16_t RF24_getSendingRSSI(void)
RF24_getSendingRSSI.
LOCAL void RF24_setAddressWidth(const uint8_t addressWidth)
RF24_setAddressWidth.
LOCAL uint8_t RF24_getFIFOStatus(void) __attribute__((unused))
RF24_getFIFOStatus.
LOCAL void RF24_setRFConfiguration(const uint8_t configuration)
RF24_setRFConfiguration.
LOCAL void RF24_setRetries(const uint8_t retransmitDelay, const uint8_t retransmitCount)
RF24_setRetries.
LOCAL bool RF24_initialize(void)
RF24_initialize.
LOCAL uint8_t RF24_getTxPowerPercent(void)
RF24_getTxPowerPercent.
LOCAL void RF24_enableFeatures(void)
RF24_enableFeatures.
LOCAL void RF24_sleep(void)
RF24_sleep.
struct @4::@5 __attribute__
Doxygen will complain without this comment.
LOCAL uint8_t RF24_getObserveTX(void)
RF24_getObserveTX.
LOCAL void RF24_openWritingPipe(const uint8_t recipient)
RF24_openWritingPipe.
LOCAL void RF24_setDynamicPayload(const uint8_t pipe)
RF24_setDynamicPayload.
LOCAL void RF24_flushTX(void)
RF24_flushTX.
LOCAL void RF24_startListening(void)
RF24_startListening.
LOCAL uint8_t RF24_spiMultiByteTransfer(const uint8_t cmd, uint8_t *buf, const uint8_t len, const bool readMode)
RF24_spiMultiByteTransfer.
LOCAL uint8_t RF24_RAW_writeByteRegister(const uint8_t cmd, const uint8_t value)
RF24_RAW_writeByteRegister.
LOCAL void RF24_setPipeAddress(const uint8_t pipe, uint8_t *address, const uint8_t addressWidth)
RF24_setPipeAddress.
LOCAL uint8_t RF24_getFeature(void)
RF24_getFeature.
LOCAL bool RF24_setTxPowerPercent(const uint8_t newPowerPercent)
RF24_setTxPowerPercent.
LOCAL void RF24_setPipe(const uint8_t pipe)
RF24_setPipe.
LOCAL bool RF24_isDataAvailable(void)
RF24_isDataAvailable.
LOCAL void RF24_setChannel(const uint8_t channel)
RF24_setChannel.
LOCAL void RF24_setPipeLSB(const uint8_t pipe, const uint8_t LSB)
RF24_setPipeLSB.
LOCAL void RF24_setNodeAddress(const uint8_t address)
RF24_setNodeAddress.
LOCAL void RF24_disableConstantCarrierWave(void) __attribute__((unused))
Stop generating a constant carrier wave (for testing only).
LOCAL void RF24_setAutoACK(const uint8_t pipe)
RF24_setAutoACK.
LOCAL void RF24_standBy(void)
RF24_standBy.
LOCAL uint8_t RF24_setStatus(const uint8_t status)
RF24_setStatus.
LOCAL void RF24_flushRX(void)
RF24_flushRX.
LOCAL uint8_t RF24_readMessage(void *buf)
RF24_readMessage.
LOCAL int16_t RF24_getTxPowerLevel(void)
RF24_getTxPowerLevel.
LOCAL bool RF24_sanityCheck(void)
RF24_sanityCheck.
LOCAL void RF24_csn(const bool level)
RF24_csn.
LOCAL uint8_t RF24_spiByteTransfer(const uint8_t cmd)
RF24_spiByteTransfer.