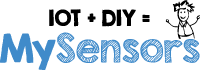 |
MySensors Library & Examples
2.3.2
|
Go to the documentation of this file.
200 #ifndef MyTransport_h
201 #define MyTransport_h
203 #include "hal/transport/MyTransportHAL.h"
205 #ifndef MY_TRANSPORT_MAX_TX_FAILURES
206 #if defined(MY_REPEATER_FEATURE)
207 #define MY_TRANSPORT_MAX_TX_FAILURES (10u)
209 #define MY_TRANSPORT_MAX_TX_FAILURES (5u)
213 #ifndef MY_TRANSPORT_MAX_TSM_FAILURES
214 #define MY_TRANSPORT_MAX_TSM_FAILURES (7u)
217 #ifndef MY_TRANSPORT_TIMEOUT_FAILURE_STATE_MS
218 #define MY_TRANSPORT_TIMEOUT_FAILURE_STATE_MS (10*1000ul)
220 #ifndef MY_TRANSPORT_TIMEOUT_EXT_FAILURE_STATE_MS
221 #define MY_TRANSPORT_TIMEOUT_EXT_FAILURE_STATE_MS (60*1000ul)
223 #ifndef MY_TRANSPORT_STATE_TIMEOUT_MS
224 #define MY_TRANSPORT_STATE_TIMEOUT_MS (2*1000ul)
226 #ifndef MY_TRANSPORT_CHKUPL_INTERVAL_MS
227 #define MY_TRANSPORT_CHKUPL_INTERVAL_MS (10*1000ul)
229 #ifndef MY_TRANSPORT_STATE_RETRIES
230 #define MY_TRANSPORT_STATE_RETRIES (3u)
234 #define BROADCAST_ADDRESS (255u)
235 #define DISTANCE_INVALID (255u)
236 #define MAX_HOPS (254u)
237 #define INVALID_HOPS (255u)
238 #define MAX_SUBSEQ_MSGS (5u)
239 #define UPLINK_QUALITY_WEIGHT (0.05f)
243 #if defined(MY_PARENT_NODE_IS_STATIC) && !defined(MY_PARENT_NODE_ID)
244 #error MY_PARENT_NODE_IS_STATIC but no MY_PARENT_NODE_ID defined!
247 #define _autoFindParent (bool)(MY_PARENT_NODE_ID == AUTO)
248 #define isValidDistance(_distance) (bool)(_distance!=DISTANCE_INVALID)
249 #define isValidParent(_parent) (bool)(_parent != AUTO)
275 void(*Transition)(void);
284 #define transportInternalToRSSI(__value) ((int16_t)__value >> 4)
285 #define transportRSSItoInternal(__value) ((transportRSSI_t)__value << 4)
310 #if defined(MY_SIGNAL_REPORT_ENABLED)
411 bool transportWait(
const uint32_t waitingMS,
const uint8_t cmd,
const uint8_t msgType);
572 #endif // MyTransport_h
void stInitUpdate(void)
Initialize transport.
bool transportSendWrite(const uint8_t to, MyMessage &message)
Send message to recipient.
uint8_t nodeId
Current node id.
void transportProcessFIFO(void)
Process all pending messages in RX FIFO.
void transportUpdateSM(void)
Update SM state.
void stReadyUpdate(void)
Monitor transport link.
void transportProcess(void)
Process FIFO msg and update SM.
bool pingActive
flag ping active
bool transportCheckUplink(const bool force=false)
Check uplink to GW, includes flooding control.
uint8_t failedUplinkTransmissions
counter failed uplink transmissions (max 15)
uint8_t transportGetParentNodeId(void)
Get parent node ID.
void transportSaveRoutingTable(void)
Save routing table to EEPROM.
uint8_t passiveMode
Passive mode.
int16_t transportSignalReport(const char command) __attribute__((unused))
Get transport signal report.
uint8_t transportGetDistanceGW(void)
Get distance to GW.
void stFailureTransition(void)
Transport failure and power down radio.
void stReadyTransition(void)
Set transport OK.
bool transportActive
flag transport active
transportState_t * currentState
pointer to current FSM state
bool transportWaitUntilReady(const uint32_t waitingMS=0)
Wait until transport is ready.
bool uplinkOk
flag uplink ok
uint8_t transportGetRoute(const uint8_t node)
Load route to node.
int16_t transportGetSignalReport(const signalReport_t signalReport) __attribute__((unused))
Get transport signal report.
uint32_t lastUplinkCheck
last uplink check, required to prevent GW flooding
uint32_t stateEnter
state enter timepoint
uint32_t transportTimeInState(void)
Request time in current SM state.
uint8_t transportGetNodeId(void)
Get node ID.
void transportTogglePassiveMode(const bool OnOff)
Toggle passive mode, i.e. transport does not wait for ACK.
bool isMessageReceived(void)
Flag valid message received.
int16_t transportRSSI_t
Datatype for internal RSSI storage.
uint8_t reserved
Reserved.
void stFailureUpdate(void)
Re-initialize transport after timeout.
void stInitTransition(void)
Initialize SM variables and transport HW.
void stParentTransition(void)
Find parent.
struct @4::@5 __attribute__
Doxygen will complain without this comment.
uint8_t pingResponse
stores I_PONG hops
void stParentUpdate(void)
Verify find parent responses.
bool transportWait(const uint32_t waitingMS, const uint8_t cmd, const uint8_t msgType)
Wait and process messages for a defined amount of time until specified message received.
void stUplinkUpdate(void)
Verify uplink response.
void transportSwitchSM(transportState_t &newState)
Switch SM state.
#define SIZE_ROUTES
Size routing table.
void transportSetRoute(const uint8_t node, const uint8_t route)
Update routing table.
void stUplinkTransition(void)
Send uplink ping request.
uint8_t transportPingNode(const uint8_t targetId)
Ping node.
void transportLoadRoutingTable(void)
Load routing table from EEPROM to RAM. Only for GW devices with enough RAM, i.e. ESP8266,...
bool findingParentNode
flag finding parent node is active
bool isTransportReady(void)
Flag transport ready.
void transportProcessMessage(void)
Receive message from RX FIFO and process.
uint32_t transportGetHeartbeat(void)
Return heart beat.
bool isTransportExtendedFailure(void)
Flag TSM extended failure.
bool transportRouteMessage(MyMessage &message)
Send and route message according to destination.
uint8_t failureCounter
counter for TSM failures (max 7)
uint8_t stateRetries
retries / state re-enter (max 7)
void transportReInitialise(void)
Reinitialise transport. Put transport to standby - If xxx_POWER_PIN set, power up and go to standby.
bool transportAssignNodeID(const uint8_t newNodeId)
Assign node ID.
void transportClearRoutingTable(void)
Clear routing table.
void resetMessageReceived(void)
Reset message received flag.
Status variables and SM state.
uint8_t parentNodeId
Where this node sends its messages.
void transportDisable(void)
Disable transport, if xxx_POWER_PIN is defined, transport is powered down, else send to sleep.
void(* transportCallback_t)(void)
Callback type.
bool msgReceived
flag message received
void stIDUpdate(void)
Verify ID response and GW link.
void transportInvokeSanityCheck(void)
Call transport driver sanity check.
void transportInitialise(void)
Initialize transport and SM.
bool preferredParentFound
flag preferred parent found
MyMessage is used to create, manipulate, send and read MySensors messages.
uint8_t distanceGW
This nodes distance to sensor net gateway (number of hops)
bool transportSendRoute(MyMessage &message)
Send and route message according to destination with transport state check.
bool isTransportSearchingParent(void)
Flag searching parent ongoing.
void transportReportRoutingTable(void)
Reports content of routing table.
void stIDTransition(void)
Send ID request.