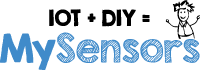 |
MySensors Library & Examples
2.3.2
|
Go to the documentation of this file.
67 #ifndef MySensorsCore_h
68 #define MySensorsCore_h
77 #define GATEWAY_ADDRESS ((uint8_t)0)
78 #define NODE_SENSOR_ID ((uint8_t)255)
79 #define MY_CORE_VERSION ((uint8_t)2)
80 #define MY_CORE_MIN_VERSION ((uint8_t)2)
82 #define MY_WAKE_UP_BY_TIMER ((int8_t)-1)
83 #define MY_SLEEP_NOT_POSSIBLE ((int8_t)-2)
84 #define INTERRUPT_NOT_DEFINED ((uint8_t)255)
85 #define MODE_NOT_DEFINED ((uint8_t)255)
86 #define VALUE_NOT_DEFINED ((uint8_t)255)
87 #define FUNCTION_NOT_SUPPORTED ((uint16_t)0)
140 bool present(
const uint8_t sensorId,
const mysensors_sensor_t sensorType,
141 const char *description =
"",
142 const bool requestEcho =
false);
143 #if !defined(__linux__)
144 bool present(
const uint8_t childSensorId,
const mysensors_sensor_t sensorType,
145 const __FlashStringHelper *description,
146 const bool requestEcho =
false);
158 bool sendSketchInfo(
const char *name,
const char *version,
const bool requestEcho =
false);
159 #if !defined(__linux__)
160 bool sendSketchInfo(
const __FlashStringHelper *name,
const __FlashStringHelper *version,
161 const bool requestEcho =
false);
228 bool request(
const uint8_t childSensorId,
const uint8_t variableType,
256 void saveState(
const uint8_t pos,
const uint8_t value);
273 void wait(
const uint32_t waitingMS);
311 int8_t
sleep(
const uint32_t sleepingMS,
const bool smartSleep =
false);
323 int8_t
sleep(
const uint8_t interrupt,
const uint8_t mode,
const uint32_t sleepingMS = 0,
338 int8_t
sleep(
const uint8_t interrupt1,
const uint8_t mode1,
const uint8_t interrupt2,
339 const uint8_t mode2,
const uint32_t sleepingMS = 0,
const bool smartSleep =
false);
359 int8_t
smartSleep(
const uint8_t interrupt,
const uint8_t mode,
const uint32_t sleepingMS = 0);
372 int8_t
smartSleep(
const uint8_t interrupt1,
const uint8_t mode1,
const uint8_t interrupt2,
373 const uint8_t mode2,
const uint32_t sleepingMS = 0);
controllerConfig_t controllerConfig
Controller config.
bool nodeRegistered
Flag node registered.
uint8_t type
8 bit - Type varies depending on command
MySensors specific configuration flags.Set these in your sketch before including MySensors....
MyMessage & setSensor(const uint8_t sensorId)
Set which child sensor this message belongs to.
bool sendSketchInfo(const char *name, const char *version, const bool requestEcho=false)
void receiveTime(uint32_t) __attribute__((weak))
Callback for incoming time messages.
bool _sendRoute(MyMessage &message)
Sends message according to routing table.
#define NODE_SENSOR_ID
Node child is always created/presented when a node is started.
void _checkNodeLock(void)
Check node lock status and prevent node execution if locked.
uint8_t sensor
8 bit - Id of sensor that this message concerns.
bool sendTXPowerLevel(const uint8_t level, const bool requestEcho=false)
void receive(const MyMessage &) __attribute__((weak))
Callback for incoming messages.
uint32_t getSleepRemaining(void)
@ C_INTERNAL
Internal MySensors messages (also include common messages provided/generated by the library).
void loop(void) __attribute__((weak))
Main loop.
int8_t _sleep(const uint32_t sleepingMS, const bool smartSleep=false, const uint8_t interrupt1=INTERRUPT_NOT_DEFINED, const uint8_t mode1=MODE_NOT_DEFINED, const uint8_t interrupt2=INTERRUPT_NOT_DEFINED, const uint8_t mode2=MODE_NOT_DEFINED)
MyMessage & setSender(const uint8_t senderId)
Set sender ID.
Controller configuration.
bool presentationSent
Flag presentation sent.
void _begin(void)
Node initialisation.
bool sendHeartbeat(const bool requestEcho=false)
uint8_t loadState(const uint8_t pos)
uint8_t destination
8 bit - Id of destination node
mysensors_command_t
The command field (message-type) defines the overall properties of a message.
MyMessage & setType(const uint8_t messageType)
Set message type.
void presentation(void) __attribute__((weak))
Node presentation.
controllerConfig_t getControllerConfig(void)
void saveState(const uint8_t pos, const uint8_t value)
struct @4::@5 __attribute__
Doxygen will complain without this comment.
bool send(MyMessage &msg, const bool requestEcho=false)
void before(void) __attribute__((weak))
Called before node initialises.
bool request(const uint8_t childSensorId, const uint8_t variableType, const uint8_t destination=GATEWAY_ADDRESS)
void _infiniteLoop(void)
Puts node to a infinite loop if unrecoverable situation detected.
bool present(const uint8_t sensorId, const mysensors_sensor_t sensorType, const char *description="", const bool requestEcho=false)
MyMessage & setEcho(const bool echo)
Setter for echo-flag.
uint8_t getParentNodeId(void)
void setup(void) __attribute__((weak))
Called after node initialises but before main loop.
MyMessage & setDestination(const uint8_t destinationId)
Set final destination node id for this message.
bool requestTime(const bool requestEcho=false)
API and type declarations for MySensors messages.
void wait(const uint32_t waitingMS)
int8_t sleep(const uint32_t sleepingMS, const bool smartSleep=false)
void _registerNode(void)
Handles registration request.
void _nodeLock(const char *str)
Lock a node and transmit provided message with 30m intervals.
Eeprom addresses for MySensors library data.
MyMessage & setCommand(const mysensors_command_t command)
Setter for command type.
bool sendBatteryLevel(const uint8_t level, const bool requestEcho=false)
bool _processInternalCoreMessage(void)
Processes internal core message.
MyMessage & setRequestEcho(const bool requestEcho)
Setter for echo request.
#define MODE_NOT_DEFINED
_sleep() param: no mode defined
bool sendSignalStrength(const int16_t level, const bool requestEcho=false)
#define INTERRUPT_NOT_DEFINED
_sleep() param: no interrupt defined
int8_t smartSleep(const uint32_t sleepingMS)
MyMessage is used to create, manipulate, send and read MySensors messages.
#define GATEWAY_ADDRESS
Node ID for GW sketch.
uint8_t isMetric
Flag indicating if metric or imperial measurements are used.
void _process(void)
Main framework process.
void preHwInit(void) __attribute__((weak))
Called before any hardware initialisation is done.